Technical interviews are a critical component of the hiring process for engineering roles. They are designed to assess not only your technical skills but also your problem-solving abilities, analytical thinking, and sometimes even your creativity. Preparing thoroughly can significantly boost your chances of success. Here’s a comprehensive guide to help you navigate the preparation process effectively.
1. Understand the Interview Structure
Understanding the structure of technical interviews is crucial for effective preparation. Here’s a detailed look at the different components and types of technical interviews you might encounter:
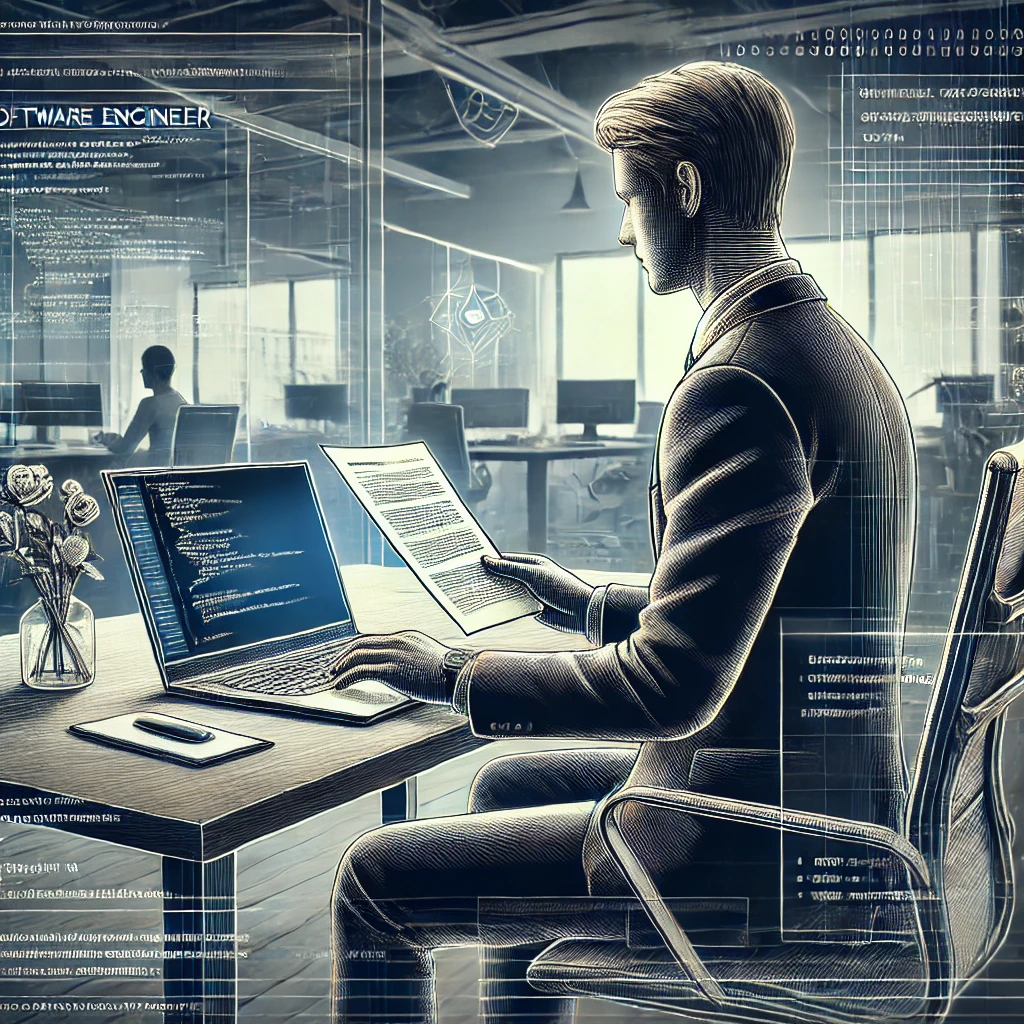
Types of Technical Interviews
- Coding/Programming Interviews:
- Purpose: These technical interviews assess your problem-solving skills and coding proficiency. You’ll be given problems that require you to write code to solve specific challenges.
- Common Topics:
- Algorithms: Sorting, searching, dynamic programming, recursion, and graph algorithms.
- Data Structures: Arrays, linked lists, stacks, queues, trees, and hash tables.
- Typical Format:
- Live Coding: You solve problems in real-time, usually on a whiteboard or coding platform. You might be asked to explain your thought process and debug your solution.
- Coding Challenges: These may be conducted on platforms like LeetCode or HackerRank, where you write and test code against various test cases.
- Example Questions:
- Implement a function to sort an array.
- Write a program to find the shortest path in a graph.
- System Design Interviews:
- Purpose: These technical interviews evaluate your ability to design complex systems and understand high-level architecture. You’ll need to demonstrate your knowledge of scalability, reliability, and maintainability.
- Common Topics:
- Scalability: Load balancing, sharding, and distributed systems.
- High Availability: Redundancy, failover mechanisms, and disaster recovery.
- APIs and Microservices: Designing RESTful APIs, understanding microservices architecture, and service communication.
- Typical Format:
- Design Questions: You might be asked to design a system like a social network, e-commerce platform, or real-time messaging service.
- Whiteboard Design: You’ll sketch out your design on a whiteboard or virtual whiteboard, explaining your choices and trade-offs.
- Example Questions:
- Design a URL shortening service (e.g., Bitly).
- Create a scalable chat application.
- Behavioral and Technical Questions:
- Purpose: These technical questions assess your overall fit for the role, including your technical background and how you handle real-world scenarios.
- Common Topics:
- Behavioral Questions: Focus on past experiences, teamwork, and problem-solving approaches. Use the STAR (Situation, Task, Action, Result) method to structure your answers.
- Technical Questions: Cover specific technical skills or knowledge related to the role. You might be asked about particular technologies, frameworks, or methodologies you’ve worked with.
- Typical Format:
- Interviews with Team Members: Discuss past projects, technical challenges, and how you’ve contributed to teams or solved problems.
- Scenario-Based Questions: Describe how you would approach hypothetical situations or technical problems.
- Example Questions:
- Tell me about a time you faced a significant challenge in a project and how you overcame it.
- How do you stay updated with the latest technologies and trends in your field?
Interview Formats
- On-site Interviews:
- Overview: These interviews take place at the company’s office and often involve multiple rounds. You might meet with several team members and go through different types of interviews.
- Typical Components:
- Technical Interviews: Coding and system design interviews with various team members.
- Behavioral Interviews: Discussions about past experiences and cultural fit.
- Team Fit: Assessments of how well you fit with the team and company culture.
- Phone/Online Interviews:
- Overview: These interviews are conducted remotely, usually via phone or video conferencing tools. They may involve coding challenges or technical discussions.
- Typical Components:
- Initial Screening: A brief interview to assess your basic qualifications and interest in the role.
- Coding Challenges: Often conducted using online coding platforms where you solve problems and share your code with the interviewer.
- Technical Discussion: Discussion of your solutions, technical skills, and problem-solving approach.
- Coding Assessments:
- Overview: Pre-interview assessments that involve solving coding problems online. They are often used as a screening tool before in-person interviews.
- Typical Components:
- Timed Challenges: Solve problems within a set time limit to demonstrate your coding skills and efficiency.
- Automated Feedback: Some platforms provide immediate feedback or test cases to validate your solutions.
Preparation Tips
- Research the Company: Understand the company’s technical interview process by checking online resources, such as Glassdoor, and reviewing any information provided by the company.
- Practice with Sample Questions: Use online resources to practice common types of technical questions you might encounter.
- Know the Role: Tailor your preparation based on the specific requirements and expectations of the role you’re applying for.
By thoroughly understanding the structure of technical interviews and preparing for each component, you can approach your interviews with confidence and increase your chances of success.
2. Brush Up on Technical Knowledge
A solid foundation in technical knowledge is crucial for excelling in technical interviews. This section outlines key areas to focus on, including data structures, algorithms, and system design concepts, along with strategies for effective learning and practice.
Data Structures and Algorithms
Data Structures:
- Arrays: Understand how arrays work, their time complexity for various operations, and common problems involving arrays, such as finding duplicates or rotating arrays.
- Linked Lists: Learn the differences between singly and doubly linked lists, and practice problems involving insertion, deletion, and reversal of linked lists.
- Stacks and Queues: Study their applications, implementations (array-based vs. linked-list-based), and common problems like balanced parentheses or implementing a queue using stacks.
- Trees: Focus on binary trees, binary search trees (BSTs), AVL trees, and heap trees. Practice traversals (in-order, pre-order, post-order) and problems involving tree manipulation and balancing.
- Graphs: Study graph representations (adjacency matrix vs. adjacency list), traversal algorithms (BFS, DFS), and shortest path algorithms (Dijkstra’s, Bellman-Ford).
- Hash Tables: Understand hash functions, collision resolution techniques (chaining, open addressing), and their applications in real-world scenarios.
Algorithms:
- Sorting Algorithms: Review common sorting algorithms such as bubble sort, selection sort, merge sort, quicksort, and heap sort. Know their time complexities and use cases.
- Searching Algorithms: Practice binary search and understand its efficiency compared to linear search. Solve problems involving search in sorted or rotated arrays.
- Dynamic Programming: Study key concepts such as memoization, tabulation, and common problems like the knapsack problem, longest common subsequence, and matrix chain multiplication.
- Greedy Algorithms: Learn how greedy approaches work and practice problems like coin change, activity selection, and Huffman coding.
- Recursion and Backtracking: Solve problems that require recursive solutions or backtracking techniques, such as generating permutations or solving the N-Queens problem.
System Design Concepts
Scalability:
- Load Balancing: Understand how load balancers distribute traffic across multiple servers and the differences between hardware and software load balancers.
- Sharding: Learn about database sharding techniques to partition data and improve performance.
- Caching: Study caching strategies (in-memory caching, distributed caching) and tools (Redis, Memcached) to optimize data retrieval.
High Availability and Reliability:
- Redundancy: Learn about redundancy techniques to ensure system reliability, such as using multiple servers or data replication.
- Failover: Understand failover mechanisms to switch to backup systems in case of failures.
- Disaster Recovery: Study strategies for recovering from catastrophic failures, including data backups and recovery plans.
APIs and Microservices:
- RESTful APIs: Familiarize yourself with REST principles, including statelessness, resource URIs, and HTTP methods (GET, POST, PUT, DELETE).
- SOAP APIs: Understand the basics of SOAP (Simple Object Access Protocol) and its use cases.
- Microservices Architecture: Learn about the principles of microservices, including service decomposition, inter-service communication, and challenges such as service discovery and data consistency.
Effective Learning and Practice
Books and Resources:
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein: A comprehensive guide on algorithms and data structures.
- “Designing Data-Intensive Applications” by Martin Kleppmann: Offers insights into system design and architecture.
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: Provides coding problems, solutions, and interview tips.
Online Courses and Tutorials:
- Coursera and edX: Offer courses on algorithms, data structures, and system design from top universities.
- Udacity and Pluralsight: Provide specialized courses on system design and software engineering.
Practice Platforms:
- LeetCode, HackerRank, CodeSignal: Platforms with a wide range of coding problems and challenges.
- System Design Primer (GitHub): A comprehensive resource for learning system design concepts and best practices.
Study Techniques:
- Practice Regularly: Solve problems consistently to build and reinforce your skills.
- Review Solutions: After solving problems, review optimal solutions and understand different approaches.
- Work on Projects: Apply your knowledge by working on personal or open-source projects to gain practical experience.
Mock Interviews and Peer Learning:
- Conduct Mock Interviews: Simulate real interview conditions with peers or mentors to get feedback and improve your performance.
- Participate in Coding Competitions: Engage in online coding competitions to test your skills and learn from others.
By brushing up on these technical areas and using effective learning strategies, you’ll be well-prepared for the technical challenges you’ll face in engineering interviews. This preparation will not only help you solve problems more effectively but also demonstrate your strong technical foundation to potential employers.
3. Practice Coding Problems
Practicing coding problems is a fundamental part of preparing for technical interviews. It helps you sharpen your problem-solving skills, become familiar with common algorithms and data structures, and gain confidence in your coding abilities. Here’s a detailed guide on how to effectively practice coding problems:
Choose the Right Platforms
1. Online Coding Platforms:
- LeetCode: Offers a wide range of problems categorized by difficulty and topic, with a focus on interview-style questions.
- Features: Problem-solving, mock interviews, and company-specific questions.
- Tips: Solve problems daily, focus on a mix of easy, medium, and hard problems.
- HackerRank: Provides coding challenges, competitions, and interview preparation kits.
- Features: Practice in various domains like algorithms, data structures, and artificial intelligence.
- Tips: Participate in contests and review solutions to learn different approaches.
- CodeSignal: Features coding challenges and assessments similar to real interview scenarios.
- Features: Arcade mode for practice, and company-specific assessments.
- Tips: Use the coding assessment tools to simulate real interview environments.
2. Other Platforms:
- CodeChef: Offers competitive programming problems and contests.
- Features: Regular contests and problem-solving practice.
- Tips: Engage in monthly contests to test your skills under time pressure.
- TopCoder: Known for competitive programming and challenging problems.
- Features: Single Round Matches (SRMs) and TopCoder Open.
- Tips: Participate in SRMs to gauge your problem-solving speed and accuracy.
Focus on Key Areas
1. Problem Solving:
- Practice Regularly: Set aside dedicated time each day to solve coding problems. Consistent practice helps improve your problem-solving skills.
- Variety of Problems: Work on a range of problems, including array manipulations, string processing, and matrix operations.
2. Time Complexity and Space Complexity:
- Understand Big O Notation: Learn to analyze the time and space complexity of your solutions.
- Optimize Solutions: Practice writing efficient code and improving the performance of your solutions.
3. Core Data Structures:
- Arrays and Strings: Practice problems involving manipulation, searching, and sorting.
- Linked Lists: Solve problems related to insertion, deletion, and reversing linked lists.
- Stacks and Queues: Work on problems like balancing parentheses and implementing queues using stacks.
- Trees and Graphs: Focus on traversals, shortest path algorithms, and tree manipulations.
4. Algorithms:
- Sorting and Searching: Implement various sorting algorithms and practice binary search.
- Dynamic Programming: Solve problems like the knapsack problem, longest common subsequence, and coin change.
- Greedy Algorithms: Practice problems involving interval scheduling and optimal coin change.
Use Effective Strategies
1. Problem Solving Approach:
- Understand the Problem Statement: Carefully read the problem description and constraints.
- Break Down the Problem: Divide the problem into smaller sub-problems or steps.
- Plan Your Approach: Outline your solution before coding. Decide on the algorithms and data structures to use.
2. Write Clean Code:
- Clarity: Write code that is easy to read and understand. Use meaningful variable names and comments.
- Modularity: Break your code into functions or methods to make it more organized and reusable.
3. Debugging:
- Test with Different Inputs: Verify your solution with a variety of test cases, including edge cases and boundary conditions.
- Use Debugging Tools: Utilize debugging tools or print statements to trace and fix issues in your code.
4. Analyze and Learn:
- Review Solutions: After solving a problem, review other users’ solutions and compare different approaches.
- Understand Mistakes: Analyze errors or inefficiencies in your solutions and learn how to improve.
Simulate Real Interview Conditions
1. Timed Practice:
- Set Time Limits: Practice solving problems within a set time limit to simulate real interview conditions.
- Focus on Speed and Accuracy: Balance the need for a correct solution with the need to solve problems quickly.
2. Mock Interviews:
- Conduct Mock Interviews: Arrange mock interviews with peers, mentors, or online platforms to simulate the interview environment.
- Get Feedback: Receive feedback on your problem-solving approach, coding style, and communication.
3. Code Reviews:
- Peer Reviews: Share your code with peers or mentors for review and feedback.
- Learn from Feedback: Incorporate suggestions and improvements into your coding practice.
Organize Your Practice
1. Track Your Progress:
- Maintain a Log: Keep track of problems you’ve solved, topics covered, and areas needing improvement.
- Set Goals: Define specific goals for each practice session, such as solving a certain number of problems or mastering a particular topic.
2. Build a Study Plan:
- Daily Routine: Establish a daily routine for coding practice, including problem-solving, reviewing concepts, and mock interviews.
- Weekly Review: Assess your progress weekly and adjust your practice plan based on your performance.
By systematically practicing coding problems and employing effective strategies, you can build the skills and confidence needed to excel in technical interviews. Regular practice, combined with thoughtful analysis and simulation of real interview conditions, will greatly enhance your problem-solving abilities and prepare you for success in your engineering interviews.
4. Study System Design
Studying system design is essential for preparing for technical interviews that focus on building scalable and efficient systems. System design interviews evaluate your ability to architect complex systems, considering aspects such as scalability, reliability, and maintainability. Here’s a detailed guide to help you prepare for system design interviews:
Key Concepts in System Design
1. Scalability:
- Horizontal vs. Vertical Scaling:
- Horizontal Scaling: Adding more machines or instances to distribute the load (e.g., adding more web servers).
- Vertical Scaling: Increasing the resources (CPU, memory) of a single machine.
- Load Balancing: Distributing incoming network traffic across multiple servers to ensure no single server becomes a bottleneck.
- Types: Round-robin, least connections, and IP hash-based load balancing.
- Sharding: Partitioning data across multiple databases or servers to handle large volumes of data and improve performance.
2. High Availability and Reliability:
- Redundancy: Implementing backup systems and components to ensure service availability even if one component fails.
- Failover: Automatic switching to a backup system when the primary system fails.
- Disaster Recovery: Strategies for recovering from catastrophic failures, including regular data backups and failover mechanisms.
3. Performance and Optimization:
- Caching: Storing frequently accessed data in memory to reduce latency and improve response times.
- Types: In-memory caching (e.g., Redis, Memcached) and distributed caching.
- Database Indexing: Creating indexes to speed up query performance.
- Query Optimization: Writing efficient queries and using database features to optimize performance.
4. Data Consistency and Integrity:
- ACID Properties: Ensuring transactions are Atomic, Consistent, Isolated, and Durable.
- Eventual Consistency: A consistency model where updates to a distributed system will eventually propagate and become consistent.
- Replication: Copying data across multiple databases or servers to ensure high availability and fault tolerance.
5. System Components and Architecture:
- Microservices: Designing systems as a collection of loosely coupled services that communicate over APIs.
- Service-Oriented Architecture (SOA): Structuring a system as a collection of services that interact through service contracts.
- APIs: Designing RESTful or SOAP APIs to allow different components or services to communicate.
Steps to Prepare for System Design Interviews
1. Understand Common System Design Patterns:
- Monolithic vs. Microservices: Learn the differences, benefits, and trade-offs between monolithic and microservices architectures.
- Event-Driven Architecture: Using events to trigger and communicate between services or components.
- Pub/Sub (Publish/Subscribe): A messaging pattern where publishers send messages and subscribers receive them.
2. Learn About Key Technologies and Tools:
- Databases:
- Relational Databases: SQL databases like MySQL, PostgreSQL. Understand normalization, indexing, and transactions.
- NoSQL Databases: Document stores (e.g., MongoDB), key-value stores (e.g., Redis), and column-family stores (e.g., Cassandra).
- Message Queues: Tools like RabbitMQ, Kafka for handling asynchronous communication between components.
- Containers and Orchestration: Using Docker and Kubernetes for containerization and managing containerized applications.
3. Practice System Design Problems:
- Design Case Studies:
- Design a URL Shortener: Consider features like URL generation, redirection, and handling large volumes of traffic.
- Design a Social Media Platform: Address user profiles, news feeds, messaging, and scalability.
- Design an E-Commerce System: Include product catalogs, user accounts, orders, and payment processing.
- Work on Real-World Scenarios: Review case studies from companies like Uber, Netflix, or Amazon to understand their system architectures.
4. Study Resources and Books:
- “Designing Data-Intensive Applications” by Martin Kleppmann: A comprehensive guide on designing scalable and reliable systems.
- “System Design Interview” by Alex Xu: Provides a framework for approaching system design interviews with real-world examples.
- “The Art of Scalability” by Martin L. Abbott and Michael T. Fisher: Focuses on scalability principles and practices.
5. Participate in Mock Interviews and Design Discussions:
- Mock Interviews: Conduct mock system design interviews with peers or mentors to practice articulating your design choices and reasoning.
- Design Discussions: Join online forums or study groups to discuss and review different system designs and approaches.
6. Build and Experiment:
- Personal Projects: Implement your own system designs or contribute to open-source projects to gain hands-on experience.
- Design and Deploy: Create and deploy small-scale versions of systems to understand real-world challenges and solutions.
Approach to System Design Interviews
1. Clarify Requirements:
- Understand the Problem: Ask questions to clarify requirements, constraints, and goals of the system you are designing.
- Define Scope: Determine the boundaries of the system and what features are essential.
2. Design and Discuss:
- Create a High-Level Architecture: Start with a high-level overview of the system, including major components and their interactions.
- Break Down Components: Dive into each component, discussing how it handles data, communicates with other components, and addresses scalability and reliability.
- Address Trade-Offs: Discuss trade-offs and justify your design decisions based on requirements and constraints.
3. Evaluate and Iterate:
- Review Scalability: Assess how your design handles increasing loads and discuss potential bottlenecks and solutions.
- Consider Reliability: Ensure your design includes mechanisms for redundancy, failover, and disaster recovery.
- Seek Feedback: Be open to feedback and iterate on your design based on suggestions and critiques.
By studying system design thoroughly and practicing with real-world scenarios, you can develop the skills needed to tackle complex design problems and perform well in system design interviews. Understanding core concepts, familiarizing yourself with key technologies, and applying your knowledge through practice will prepare you for success in designing scalable and efficient systems.
5. Behavioral Interviews Preparation
Behavioral interviews assess your past experiences, work habits, and interpersonal skills to predict how you might perform in future situations. Preparing for these interviews involves understanding common behavioral questions, using structured response techniques, and showcasing your relevant experiences effectively. Here’s a comprehensive guide to preparing for behavioral interviews:
1. Understand Common Behavioral Interview Questions
Behavioral questions typically focus on past experiences and how you handled various situations. Common questions include:
- Teamwork: “Can you describe a time when you worked on a team project? What was your role, and how did you handle conflicts or disagreements?”
- Leadership: “Tell me about a time when you led a project or team. How did you motivate your team and ensure the project’s success?”
- Problem-Solving: “Describe a challenging problem you faced at work and how you approached solving it.”
- Time Management: “Give an example of how you managed multiple priorities or deadlines. What strategies did you use to stay organized?”
- Adaptability: “Tell me about a time when you had to adapt to a significant change at work. How did you handle it?”
2. Use the STAR Method for Structuring Responses
The STAR method helps you structure your answers in a clear and concise way. It stands for:
- Situation: Describe the context or background of the situation.
- Task: Explain the specific task or challenge you faced.
- Action: Detail the actions you took to address the task or challenge.
- Result: Share the outcome or results of your actions, including any lessons learned.
Example:
- Situation: “In my previous role as a project coordinator, we faced a sudden change in project scope due to client feedback.”
- Task: “My task was to realign the project plan and communicate the changes to the team without causing delays.”
- Action: “I organized a meeting to discuss the changes, updated the project timeline, and assigned new tasks to team members.”
- Result: “As a result, we successfully integrated the changes and delivered the project on time, with positive feedback from the client.”
3. Reflect on Your Experiences
1. Identify Key Experiences:
- Professional Achievements: Think about projects, initiatives, or tasks where you made significant contributions or achieved notable results.
- Challenges and Solutions: Reflect on situations where you overcame obstacles or solved complex problems.
- Teamwork and Leadership: Consider instances where you collaborated with others or took on leadership roles.
2. Prepare Specific Examples:
- Quantify Results: Whenever possible, include specific metrics or outcomes to illustrate the impact of your actions (e.g., “increased sales by 20%”).
- Highlight Skills: Focus on key skills such as communication, problem-solving, leadership, and adaptability in your examples.
3. Practice Describing Experiences:
- Rehearse Answers: Practice answering common behavioral questions using the STAR method to build confidence and clarity.
- Seek Feedback: Share your responses with friends or mentors to get feedback and refine your answers.
4. Develop a Personal Narrative
1. Craft Your Story:
- Career Journey: Develop a cohesive narrative that highlights your career progression, key achievements, and how your experiences have prepared you for the role you’re interviewing for.
- Core Values: Identify and articulate the core values and principles that guide your work and decision-making.
2. Align with Job Requirements:
- Understand the Role: Review the job description and identify key competencies and qualities the employer is seeking.
- Tailor Your Examples: Select examples from your experiences that align with the job requirements and demonstrate your fit for the role.
5. Practice Active Listening and Communication
1. Listen Carefully:
- Understand the Question: Ensure you fully understand each question before responding. If needed, ask for clarification.
- Stay Focused: Listen actively to the interviewer’s follow-up questions or prompts.
2. Communicate Clearly:
- Be Concise: Provide clear and concise responses without unnecessary details.
- Stay Positive: Frame your experiences positively, even when discussing challenges or failures. Focus on what you learned and how you grew from the experience.
6. Prepare Questions for the Interviewer
1. Ask Insightful Questions:
- Company Culture: Inquire about the company culture and values to determine if they align with your own.
- Team Dynamics: Ask about the team you’ll be working with and the management style.
- Career Growth: Explore opportunities for professional development and career advancement within the company.
2. Show Genuine Interest:
- Research: Demonstrate that you’ve researched the company and the role by asking relevant and thoughtful questions.
- Engagement: Engage in a two-way conversation and express your enthusiasm for the position and the company.
7. Prepare for Behavioral Interviews with Mock Interviews
1. Conduct Mock Interviews:
- Simulate Real Interviews: Practice with mock interviews to simulate real interview conditions and receive feedback on your responses.
- Use Online Tools: Utilize online platforms or services that offer mock behavioral interviews and feedback.
2. Review and Improve:
- Analyze Performance: Review your performance in mock interviews to identify areas for improvement.
- Refine Responses: Adjust your answers based on feedback and practice to enhance your delivery and effectiveness.
8. Build Confidence and Manage Anxiety
1. Practice Regularly:
- Consistency: Regular practice helps build confidence and reduce anxiety. The more you practice, the more comfortable you’ll become with discussing your experiences.
2. Use Relaxation Techniques:
- Stress Management: Utilize relaxation techniques such as deep breathing, visualization, or mindfulness to manage anxiety before and during the interview.
By preparing thoroughly for behavioral interviews, you can effectively communicate your experiences, skills, and fit for the role. Understanding common questions, using structured response techniques, reflecting on your experiences, and practicing regularly will help you present yourself confidently and compellingly to potential employers.
Preparing for technical interviews in engineering requires a multifaceted approach, combining technical knowledge, practical problem-solving, and effective communication. By understanding the interview structure, brushing up on key concepts, practicing coding and system design, and simulating real interview conditions, you can significantly enhance your performance. Remember, preparation is key, and a structured approach will help you tackle interviews with confidence and skill.
Lead Your Job Search with Resumofy Take control of your job search with Resumofy’s AI-powered resume builder. Create resumes that resonate with employers, manage your applications effectively, and get valuable insights from our ML-based analysis. Also, craft personalized cover letters with our AI tool. Visit Resumofy to get started. Also Read :Making the Most of Your Personal and Professional Network for Resume Content.